How to Fetch G Suite Service Status Programatically
Here's one approach to fetching G Suite's service status since Google doesn't offer an API for it.
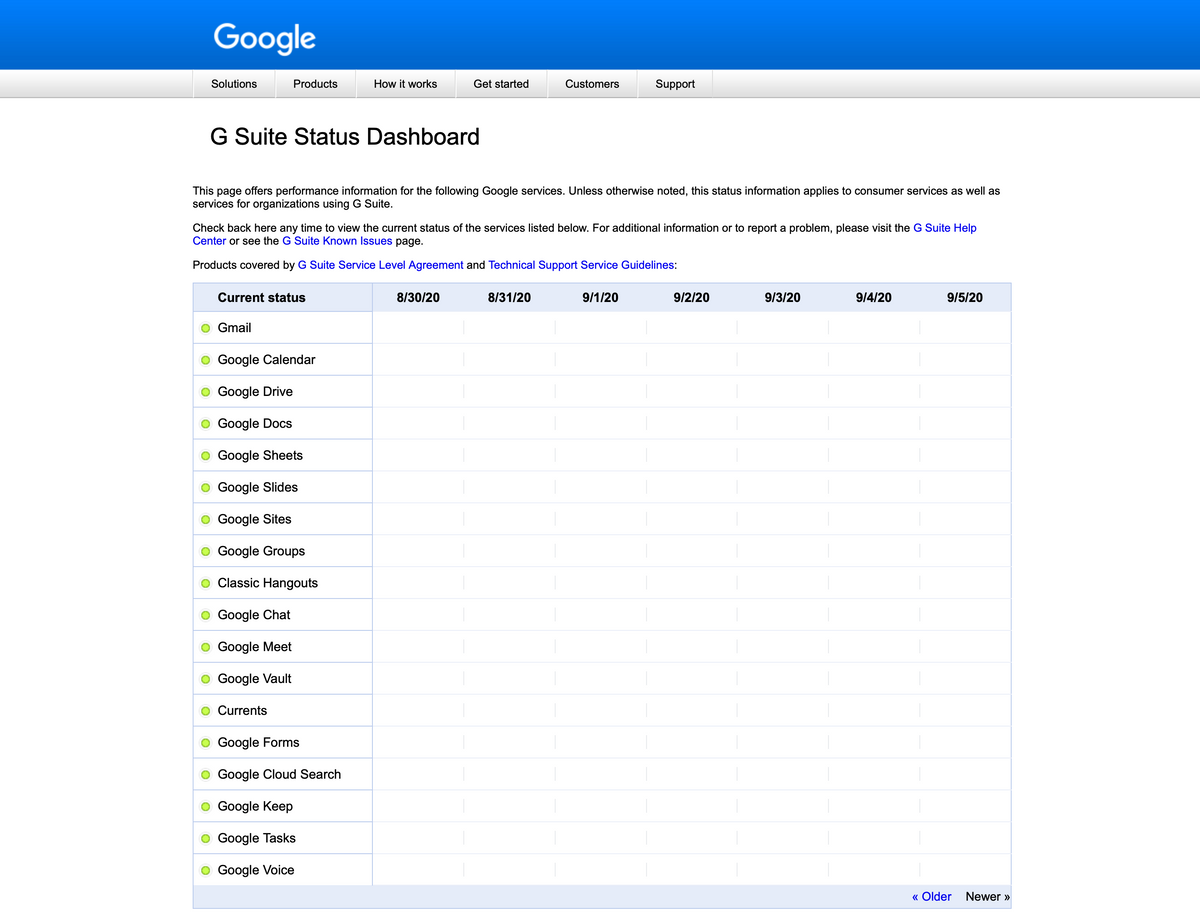
The Problem
I recently worked on a project which required the automatic fetching of the G Suite service status from the G Suite Status Dashboard page. G Suite already offers a service which sends emails to the G Suite admins if there are ongoing incidents; if that is enough for you, then there is no need to fetch the G Suite service status and hence no reason to continue reading this document. This post is intended for use cases that require a programatic way to fetch the G Suite status, since Google does not offer an API for determining if there are any issues in their service.
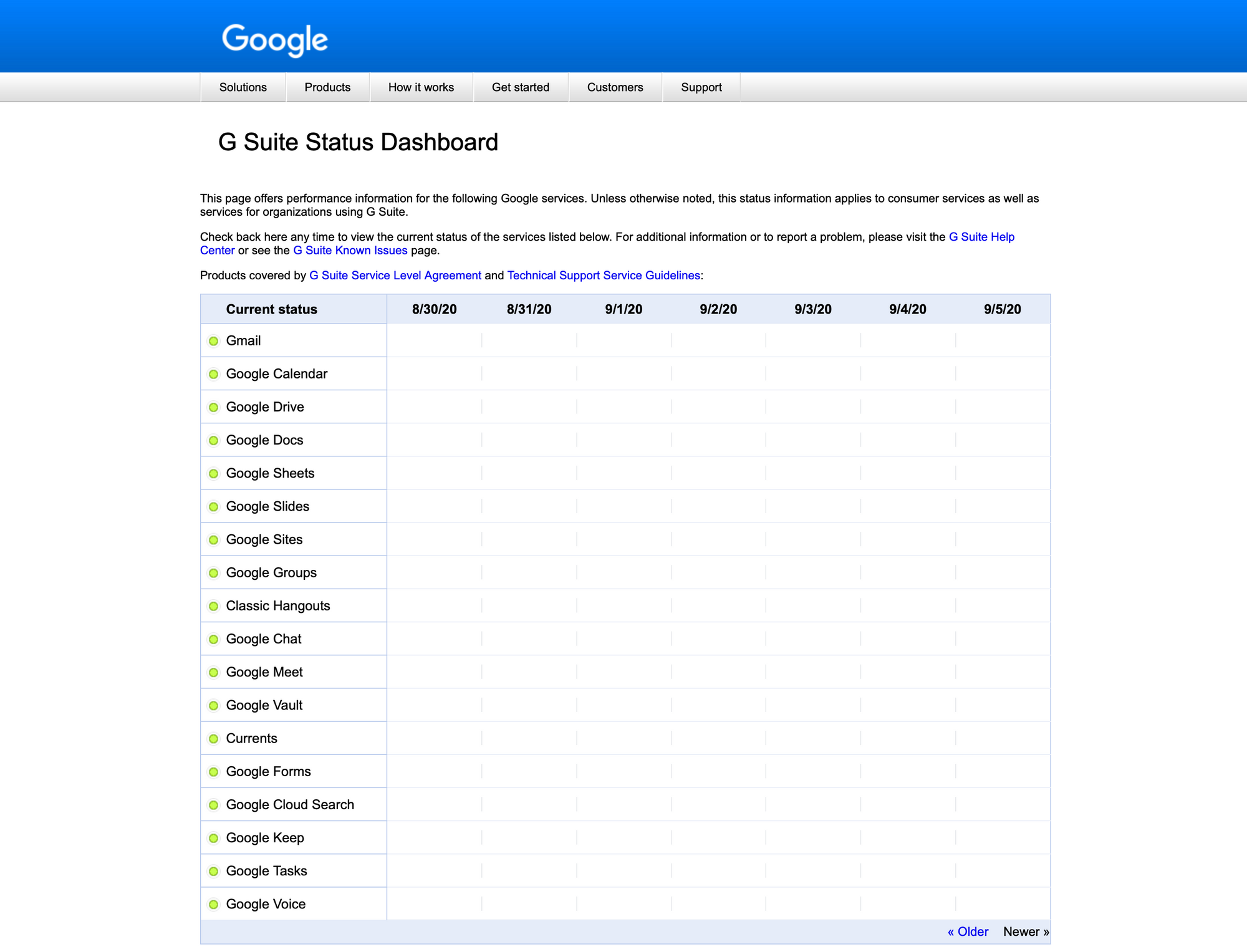
Google has made it very difficult to get the current SaaS status. While most other SaaS service status pages (e.g. Box, Zoom, Lever) offer some form of API call or webhook when the service status changes, for some reason the G Suite Status Dashboard lacks these features. When an API endpoint does not exist, a common approach for fetching service statuses from third-party websites is to parse their HTML DOM using a tool such as Jsoup; this approach, however, does not work for the G Suite Status Dashboard page because it requires JavaScript to be executed in order to see the status div's. As evidence, notice how the server returns an empty aad-content
div.
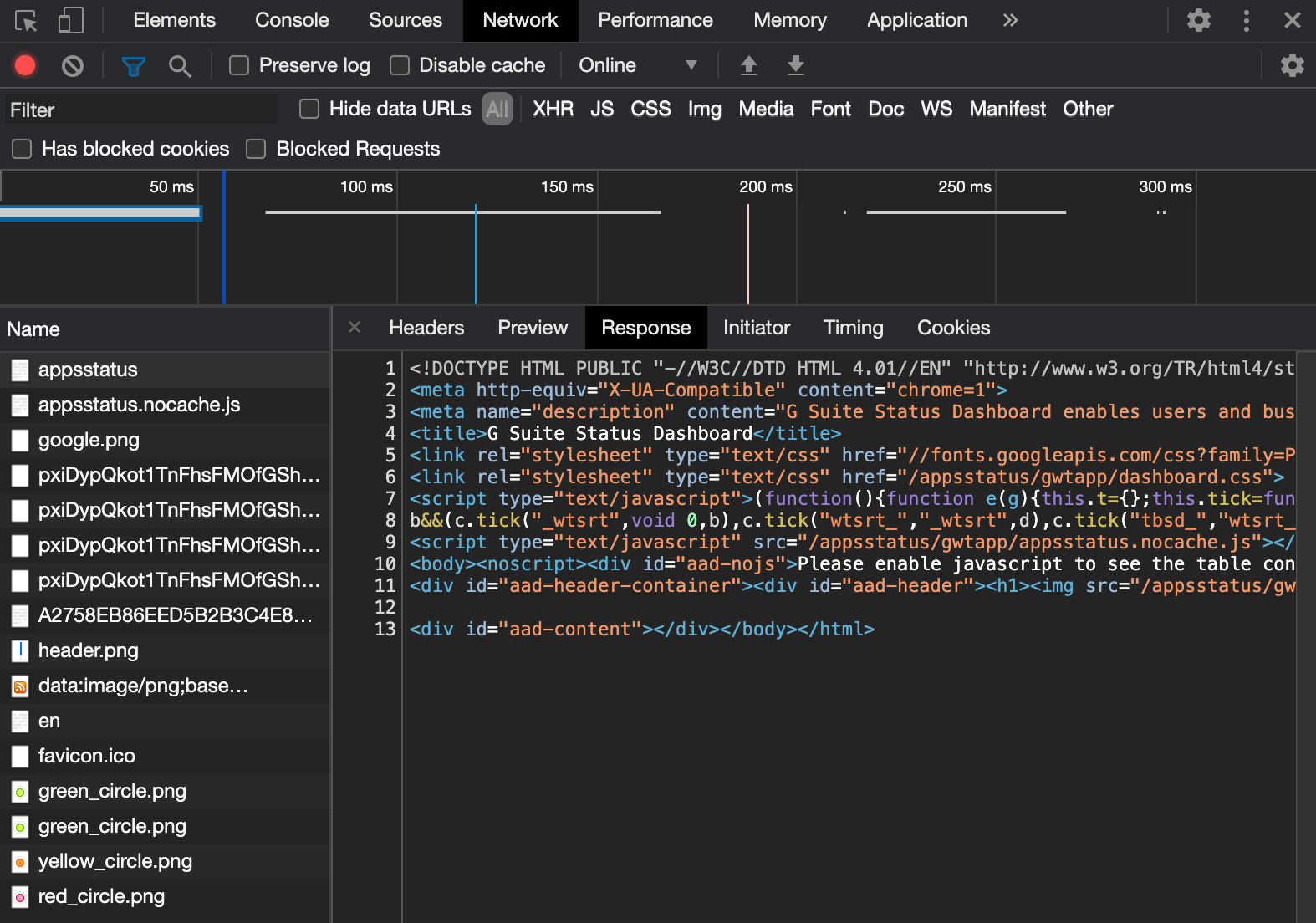
That leaves us with a few remaining options for getting the G Suite Status:
- Try to reverse-engineer an API called by the front end.
This doesn't work because it turns out that there are no HTTP calls - all of the information is passed from Google's backend servers, I think within JavaScript. I came to this conclusion by inspecting the Network tab in the Google Chrome developer tab.
2. Use a web browser automation tool like Selenium to execute the JavaScript and then parse the DOM.
This is the approach that I will discuss in the remainder of this post.
The Solution
Leaving actual code aside, here is the design for a solution to get the G Suite Status programatically.
- Use Selenium with the Chrome web driver. Selenium is a library originally designed for automated web front end testing.
- You can use this Boni Garcia's highly rated webdrivermanager library to install the correct version of the Chrome web driver which matches your installed version of Chrome.
- If your project is run on your computer, then you'll just need Chrome installed locally. If you plan to run in a Docker container and deploy using a service like Kubernetes, then make sure to add chrome installation instructions into the dockerfile. If your code runs on Ubuntu linux, here is an example of the snippet to add to your Dockerfile.
- In your code logic, use the By.cssSelector to find the status of each table row item like this. The idea is that if any of the table row status icons are red or yellow, then G Suite has some issue.
// Give the page some time to load all of the elements.
Thread.sleep(2);
// Search for the div which contains both classes `.add-body` and `.add-icon` and fetch the span child.
List<WebElement> statusIcons = new WebDriverWait(driver, Duration.ofSeconds(3).getSeconds()).until(driver -> driver.findElements(By.cssSelector(".add-body.add-icon>span")));
// Match on the classname.
for (WebElement statusIcon : statusIcons) {
String statusIconClass = statusIcon.getAttribute("class");
if (statusIconClass.contains("red") {
// G Suite outage
} else if (statusIconClass.contains("yellow") {
// G Suite service disruption
}
}
// If none of the status icons are red or yellow, then G Suite is fully operational.
5. Chances are that you won't need to see the Chrome browser, in which case you should use headless Chrome. If you are writing backend code that will run in a Docker container somewhere in the cloud, then you'll definitely want to use the headless Chrome browser. Here is a sample code snippet to configure the ChromeDriver to be headless
import org.openqa.selenium.*;
import org.openqa.selenium.chrome.*;
WebDriverManager.chromedriver().setup();
ChromeOptions options = new ChromeOptions();
options.addArguments("--no-sandbox");
options.addArguments("--disable-dev-shm-usage");
options.addArguments("--set-headless");
WebDriver driver = new ChromeDriver(options);
Conclusion
I have introduced an approach for monitoring G Suite's service status. This similar approach using Selenium can be applied to other third-party services such as OneLogin, which also has a tricky way for getting service statuses. Furthermore, this approach using Selenium can be used for many other use cases that require scraping information from a web page which requires JavaScript to be run. Feel free to ask any questions or leave comments below.